課程1 <<
Previous Next >> ANSIC
練習1
1.
/* =========================
struct – 3.
========================= */
#include <stdio.h>
#include <string.h>
/* ====================
struct Mouse.
==================== */
struct Mouse
{
int xPos, yPos;
char Name[10];
};
/* ====================
Copy Mouse 1
==================== */
void cpyMouse1(struct Mouse *tgt, struct Mouse *src)
{
tgt->xPos = src->xPos;
tgt->yPos = src->yPos;
strcpy(tgt->Name, src->Name);
}
/* ====================
main function.
==================== */
int main()
{
struct Mouse myMouse, yourMouse;
yourMouse.xPos = 10;
yourMouse.yPos = 20;
strcpy(yourMouse.Name, "Mickey");
cpyMouse1(&myMouse, &yourMouse);
printf("Name: %s, X: %d, Y: %d\n", myMouse.Name, myMouse.xPos, myMouse.yPos);
return 0;
}
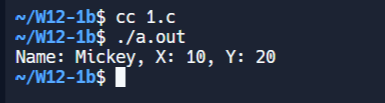
2.
/* =========================
#define 的範例 2.
========================= */
#include <stdio.h>
#define TRUE 1
#define FALSE 0
#define BOOL int
/* ====================
如果傳入值大於 10, 則傳回 TRUE
==================== */
BOOL is_greater_than_10(int i)
{
if (i > 10)
return TRUE;
else
return FALSE;
}
/* ====================
main function.
==================== */
int main()
{
int i;
BOOL result;
printf("Input a number: ");
// 檢查 scanf 的返回值
if (scanf("%d", &i) != 1) {
printf("Error reading input.\n");
return 1; // 返回非零值表示錯誤
}
result = is_greater_than_10(i);
if (result == TRUE)
printf("Greater than 10!\n"); /* 大於 10 */
else
printf("Not greater than 10!\n"); /* 不大於 10 */
return 0;
}
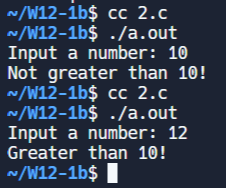
3.
/* ====================
& operator.
==================== */
#include <stdio.h>
int main()
{
int *pointer_a, a;
pointer_a = &a;
a = 10;
printf("%d, %d\n", a, *pointer_a);
return 0;
}
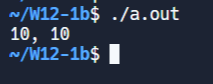
4.
/* ====================
pointer – 6
==================== */
#include <stdio.h>
int main()
{
char *str = "Eric";
printf("%c\n", *(str + 0)); /* 也可寫 printf("%c", str[0] ); */
printf("%c\n", *(str + 1)); /* 也可寫 printf("%c", str[1] ); */
printf("%c\n", *(str + 2)); /* 也可寫 printf("%c", str[2] ); */
printf("%c\n", *(str + 3)); /* 也可寫 printf("%c", str[3] ); */
return 0;
}
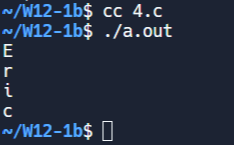
5.
/* ====================
2d array.
==================== */
#include <stdio.h>
int main()
{
int array[3][3];
int x, y;
array[0][0] = 1;
array[0][1] = 2;
array[0][2] = 3;
array[1][0] = 4;
array[1][1] = 5;
array[1][2] = 6;
array[2][0] = 7;
array[2][1] = 8;
array[2][2] = 9;
for (x = 0; x < 3; x++)
{
for (y = 0; y < 3; y++)
{
printf("%d,", array[x][y]);
}
}
return 0;
}
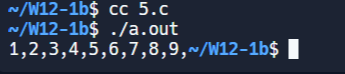
6.
/* ====================
array - 1.
==================== */
#include <stdio.h>
int main()
{
int grade[5]; /* size = 5 的 array */
int i;
grade[0] = 75; /* 1st element */
grade[1] = 80; /* 2nd element */
grade[2] = 85; /* 3rd element */
grade[3] = 70; /* 4th element */
grade[4] = 90; /* 5th element */
for (i = 0; i < 5; i++)
{
printf("Number %d = %d\n", i, grade[i]);
}
return 0;
}
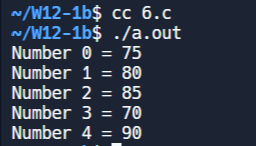
7.
/* ====================
do - while.
==================== */
#include <stdio.h>
int main()
{
int i, j;
i = 0;
j = 10; /* 迴圈外先設定初值 */
do
{
printf("i = %d, ", i);
printf("j = %d\n", j);
i++;
j++;
} while (i < 6); /* 檢查條件的地方 */
return 0;
}
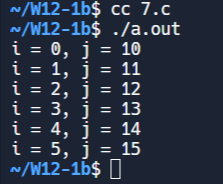
8.
/* ====================
99 乘法.
==================== */
#include <stdio.h>
int main()
{
int x, y;
for (x = 1; x <= 9; x++)
{
for (y = 1; y <= 9; y++)
{
printf("%2d ", x * y); /* 使用 %2d 以確保數字的對齊性 */
}
printf("\n");
}
return 0;
}
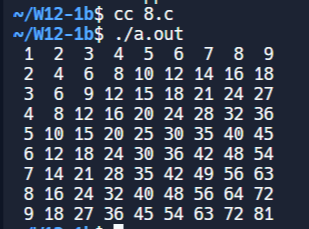
9.
/* ====================
Program "continue"
==================== */
#include <stdio.h>
int main()
{
int i;
for (i = 0; i < 10; i++)
{
if ((i == 1) || (i == 2) || (i == 3))
{
continue; /* 忽略以後的 program, 回到 for. */
}
printf("i = %d\n", i);
}
return 0;
}
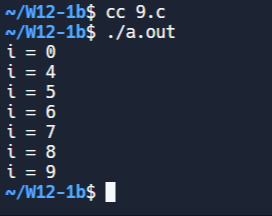
10.
/* ====================
switch - case 的範例 3.
==================== */
#include <stdio.h>
int main()
{
char c;
printf("Input a char: ");
// 檢查 scanf 的返回值
if (scanf("%c", &c) != 1)
{
printf("Error reading input.\n");
return 1; // 返回非零值表示錯誤
}
switch (c)
{
case 'a':
printf("You pressed a\n");
break;
case 'b':
printf("You pressed b\n");
break;
case 'c':
printf("You pressed c\n");
break;
default:
printf("Unrecognized input\n");
break;
}
return 0;
}
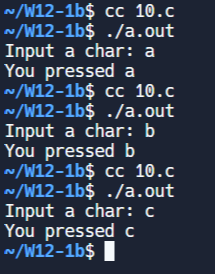
課程1 <<
Previous Next >> ANSIC